mongodb常见查询操作符的简单操作。
为了测试用,先在myTest库下建一个goods集合。use goods ,然后往里面插入一组数据。
如:
var goods = [{ "productId":"10001", "productName":"小米6", "price":"1499", "prodcutImg":"mi6.jpg" }, { "productId":"10002", "productName":"小米笔记本", "price":"4999", "prodcutImg":"note.jpg" }, { "productId":"10003", "productName":"小米7", "price":"5499", "prodcutImg":"mi6.jpg" }, { "productId":"10004", "productName":"note3", "price":"2499", "prodcutImg":"1.jpg" }, { "productId":"10005", "productName":"小米9", "price":"3499", "prodcutImg":"2.jpg" }, { "productId":"10006", "productName":"小米6", "price":"2999", "prodcutImg":"3.jpg" }, { "productId":"10007", "productName":"小米8", "price":"2499", "prodcutImg":"4.jpg" }, { "productId":"10008", "productName":"红米", "price":"999", "prodcutImg":"5.jpg" }] db.goods.insert(goods)
接下来简单查询想要数据
一些操作符简单说明:
1、$gt -------- 大于 > 2、$gte ------- 大于等于 >= 3、$lt -------- 小于 < 4、$lte ------- 小于等于 <= 5、$eq ------- 等于 = 6、$ne -------- 不等于 != 8、$type 可以用来根据数据类型查找数据 9、$and 逻辑与操作符 db.goods.find({$and:[{'price':{$gt:100}},{'price':{$lt:200}}]}) 10、$or 逻辑或操作符 db.goods.find({$or:[{'price':{$gt:100}},{'price':{$lt:200}}]}) 11、$not 取反操作符 比如查询字段price的类型不为数字的所有文档 db.goods.find({'price':{$not:{$type:1}}}) 12、$nor 无对应项, 指"所有列举条件都不成功则为真"。 {$nor,[条件1,条件2]}
$in 表示查询某一个字段在某一个范围中的所有文档,比如我想查询字段price为100或者200的所有文档,如下:
db.goods.find({'price':{$in:[100,200]}})$nin的作用刚好和$in相反,表示查询某一个字段不在某一个范围内的所有文档,比如我想查询字段price不为100或者不为200的所有文档,如下:
db.goods.find({'price':{$nin:[100,200]}})
其他操作符说明查看:
1、查询id为10008的商品
db.goods.find({'productId':10008})
如图成功查出来了
2、查询价格大于3000的商品,并且只显示price,productName属性 (pretty()-格式化显示查询结果)
db.goods.find({'price':{$gt:'3000'}},{'price':1,'_id':0,'productName':1}).pretty()
3、查询价格大于3000且小于5000的商品 $and 、$gt 、 $lt
db.goods.find({$and:[{'price':{$gt:'3000'}},{'price':{$lt:'5000'}}]}).pretty()
db.goods.find({'productId':{$in:['10004','10008']}},{'productId':1,'price':1}).pretty()
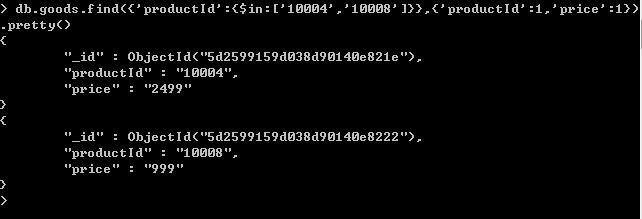
5、查询不属于第4栏目且不属于第8栏目的商品
第一种用 $and:
db.goods.find({$and:[{'productId':{$ne:'10004'}},{'productId':{$ne:'10008'}}]}).pretty()第二种用 $nin:
db.goods.find({'productId':{$nin:['10004','10008']}}).pretty()第三种用 $nor:
db.goods.find({$nor:[{'productId':'10004'},{'productId':'10008'}]}).pretty()
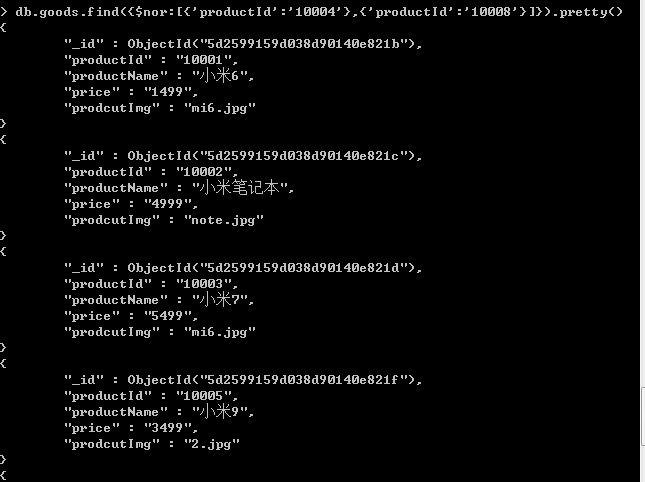
6、查询id不等于10001的所有商品 $not
db.goods.find({'productId':{$not:'10001'}}).pretty()7、数据分页可以使用 limit与skip方法
limit()方法接受一个数字参数,该参数指定从MongoDB中读取的记录条数。
skip()方法同样接受一个数字参数作为跳过的记录条数。
比如我们goods开始插入的是8条数据,如果分两页,每页4条。我们要查第二页的数据,可以这样:
db.goods.find({}).limit(4).skip(4).pretty()
……
MongoDB学习记录-索引的简单操作(三)